executeScript - Selenium IDE
executeScript (JS code, variable) - Selenium IDE command
executeScript
The executeScript command executes a snippet of JavaScript in the context of the currently selected frame or window. The script fragment will be executed as the body of an anonymous function. To store the return value, use the 'return' keyword and provide a variable name in the value input field.
executeScript_Sandbox
The executeScript_Sandbox works in principle as executeScript but runs the Javascript in a sandbox. In other words, the code runs not in the website. The advantage of using the sandbox is that the website can not influence or block the Javascript execution. So unless you want to access elements of the website or need to use ES6+ features, better use the _sandbox version of the command. The flow control commands (if, while, GotoIf) use executeScript_Sandbox internally to evaluate the expression.
A good example is the Github.com website. If that website is loaded, executeScript (without sandbox) fails with errors such as "Call to eval() blocked by CSP". CSP stands for (Chrome) Content Security Policy error. The website blocks the Javascript execution. But executeScript_Sandbox works just fine, as the Javascript is not executed inside the website.
Changes with RPA Version 7.x
As of Ui.Vision RPA V7.0 executeScript_Sandbox is no longer 100% compatible with executeScript. The reason is that due to the upgrade to the new Chrome manifest V3 we must use different Javascript interpreter for the sandboxed version. So while executeScript (which runs in the website) remains unchanged, the _Sandbox version uses now JS-Interpreter.
For simple Javascript there is no difference. But some more advanced commands are missing in _Sandbox because the JS-interpreter only supports ES5 level Javascript (and not ES6 yet). For example, instead of "const" you need to use "var". Another example is that instead of ".includes" use ".indexof".
Arrays in Selenium IDE
Arrays in Selenium IDE are Javascript arrays. To create a Javascript array use the executeScript_Sandbox command (see forum post). To loop over an array use the for each command.
Useful Javascript snippets for use with executeScript
Use them like executeScript | Javascript here | VarWithResult. Then later in the macro, you can use the result with the ${VarWithResult} variable.
All example snippets in the first table work fine in the sandbox. We recommend to use executeScript_Sandbox, but executeScript will work as well.
Javascript (executeScript_Sandbox recommended) | Result |
---|---|
return Math.floor(Math.random()*11) | Random number between 0 and 10 |
return Number (${i}) + 1; | Increase the value of i by one (very useful inside loops) |
var x="abc"; return x.length; | Returns the length of the string (here 3). |
x="${myvar}"; return x.length; | Same as above but with variable as input. |
var d=new Date(); return d.getDate()+'-'+((d.getMonth()+1))+'-'+d.getFullYear(); | Get todays date |
var d= new Date(); var m=((d.getMonth()+1)<10)?'0'+(d.getMonth()+1):(d.getMonth()+1); return d.getFullYear()+"-"+m+"-"+d.getDate(); | Get todays date in YYYY-MM-DD format |
var d= new Date(); var month = d.toLocaleString('default', { month: 'long' }); return month+"-"+d.getDate() + "-"+ d.getFullYear(); | Get todays date in MMM-DD-YYYY where MMM is the month's name e. g. May-1-2022 |
var d= new Date(new Date().getTime() + 24 * 60 * 60 * 1000 * 5); var m=((d.getMonth()+1)<10)?'0'+(d.getMonth()+1):(d.getMonth()+1); m=d.getFullYear()+"-"+m+"-"+d.getDate(); return m | Get yesterday's date (-1), tomorrows date (1) or the date in 5 days (5) => Just change the number 5 in the code snippet. |
return parseFloat(${!runtime})-parseFloat(${starttime}); | Calculate the difference between the current runtime and the start time. Very useful for measuring website performance. !runtime is a built-in variable, and starttime is a regular variable, we can fill it with !runtime e. g. at the macro start. |
var x = parseInt((new Date('2018-03-20T12:00:00') - new Date()) / 1000); return Math.max(0, x); | Countdown to specific date and time. Use the result as input for PAUSE |
return ["Hello","Bonjour","你好"]; | Create a Javascript array. You can use for Each to loop over the array. |
The examples below all interact with the website, that is why we need to use executeScript.
Javascript (executeScript only) | Result |
---|---|
return window.document.getSelection().toString() | Copy selected text (e. g. text marked with Ctrl+A) |
alert("Hello World!") | Show an alert box. [Needs website] Note: Since Chrome 64 the prompt box is only shown (by Chrome) if the tab has the focus. This is a change in Chrome, not Kantu. Better use PAUSE | 0 |
return prompt("Please enter a value") | Ask user for input and store it in a variable. Note: Since Chrome 64 the prompt box is only shown (by Chrome) if the tab has the focus. This is a change in Chrome, not Kantu. Better use the internal PROMPT command of Kantu. |
return window.document.getElementsByName('Phone')[0].value; | Retrieve the field value an input box. This is also a replacement for storeValue from the old IDE. storeAttribute does not work with input boxes. |
return document.getElementByClassName(“label-bold”)[0].innerHTML; | Extract some data, in this case from "<div class=“label-bold”> Order Number# 123456 </div>" |
window.history.go(-1); | Simulate BACK button click |
var audio = new Audio('http://www.storiesinflight.com/html5/audio/shimmer.wav');audio.play(); | Play sound! |
execute Script Example
This example calculates 100-20, and then sets the page title to the result (80).
Command | Target | Pattern/Text |
---|---|---|
open | https://ui.vision/ | |
store | 100 | Var1 |
store | 20 | Var2 |
executeScript_Sandbox | return Number (${Var1}) - Number (${Var2}) | Var3 |
executeScript | document.title = ${Var3}; | |
assertTitle | 80 |
Works in
Ui.Vision RPA for Chrome Selenium IDE, Ui.Vision RPA for Firefox Selenium IDE, Firefox IDE Classic
Related Demo Macros
DemoExecuteScript, DemoIfElse, DemoCsvReadArray
The ready-to-import-and-run source code of all demo macros can be found in the Open-Source RPA software Github repository.
See also
executeAsyncScript, storetext, storetitle, Web Automation Extension User Manual, Selenium IDE commands, Classic Firefox Selenium IDE.
Anything wrong or missing on this page? Suggestions?
...then please contact us.
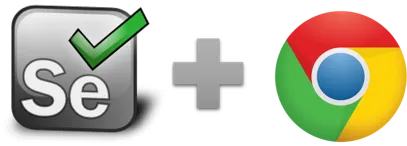